Part 2: A Beginners’ guide to WordPress REST API
Are you wondering how to start working with WordPress REST API? This simple tutorial for programmers with no REST API experience might just be the answer to those questions. In the article, you will learn how to work with Postman, what are endpoints and routes, how to authenticate yourself and how to communicate with REST API in practice.
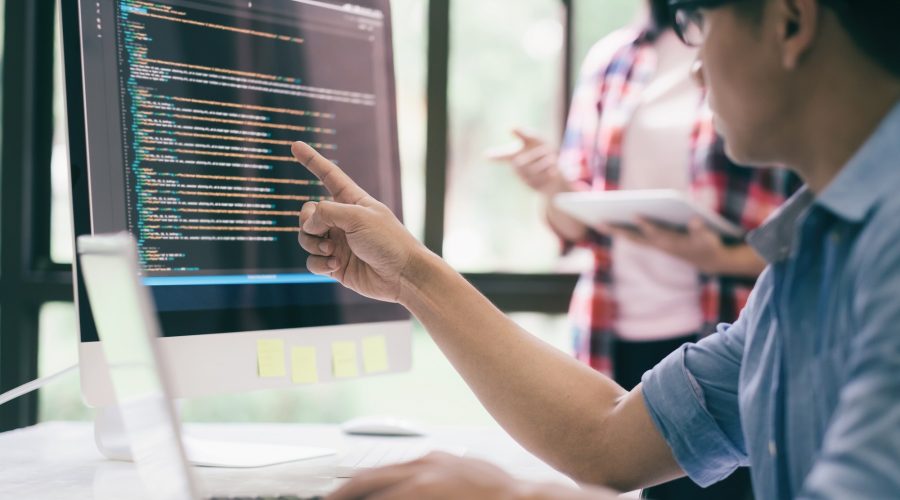
If you are starting with REST API and want to work with it in your project, you’re in the right place. In the article, we will introduce you the most important concepts of the REST API and guide you through the first operations step by step.
If you want to know more general information about REST API and its benefits, read the first part of the series WordPress REST API – what it can do and how can it be of use to you.
Starting with Postman
First, in order to try out the communication with REST API, you need to install WordPress – have web hosting with PHP or a configured local web server with a WordPress installation on it. You can find more information about the installation in the official WordPress documentation. For full employment of WP REST API, you’ll need the WordPress version 4.7 and newer.
Postman is a native application for Windows, OS X, and Linux to design and communicate with REST API.
For testing purposes, it is necessary to install an HTTP client to send requests and display responses. The Postman can be such a client. Postman shows what a server request should look like. You define a method and endpoint, a body of the request, add authentication data and send all this information. Afterward, you receive a response from the server. You can use the URL https://postman-echo.com as a testing server, there are a few testing requests as well.
However, in a real project, the Postman won’t be enough. You’ll have to work with libraries for communication via HTTP protocol. In PHP, it is library cURL (you’ll find the example with cURL at the end of the article).
Key concepts
Authentication
The basic concept in REST API is the authentication. You can’t work with API without authenticating yourself. Simply put – authentication is a process of proving your identity to a server to be able to send requests. Once you prove your identity, there is no obstacle in front of you in deleting posts or receiving data about users. For that reason, it is necessary to authenticate before each use of the program.
REST API enables more options of authentication, each type is better suited to a different occasion. However, authentication would be a topic of a separate extensive article so we will only present a brief summary of authentication options. You can find specific information in the official documentation in the section Authentication.
- Basic authentication sends the name and password of a user in the head of the request. It is not safe enough so it is useful only for testing purposes.
- Next option is based on the basic authentication as well (so-called application password), although it uses a key generated by the server to be inserted to a client instead of a name and password. This variant requires a WordPress plugin.
- Authentication via cookies is utilized for communication in JavaScript in the WordPress framework between an interface with a browser and a server.
- The OAuth authentication is the most sophisticated variant which is used by various services (Google, Twitter). The user proves his identity out of the application, the key is provided to him and he uses it to log in.
In Postman, options of authentication are presented under different names. You can find more information about authentication in Postman here.
HTTP protocol and CRUD operations
Communication in the application is executed via HTTP protocol. Our application creates a request and sends it to a WordPress server with API. The address to which a request is routed is called an endpoint (we explain it later).
REST API performs the so-called CRUD operations based on HTTP protocol. API makes use of a limited set of HTTP request verbs with defined meaning which are as following:
- GET – used to read or retrieve a resource
- POST – used to create a new resource
- PUT – used to update a resource
- DELETE – used to delete a resource
- HEAD – used to check if a resource exists
- OPTIONS – used to retrieve all the verbs supported by a resource
The first four verbs are components of CRUD actions, the last two requests assist a client in determining whether a resource exists and what HTTP verbs can be used for executing further operations.
HTTP responses
A server with WordPress installation responds to a request by returning a response comprised from the HTTP status code and data in JSON format. Status codes are numbers with predefined meanings associated with them. All web users probably know the 404 status code – that what the user was looking for was not found. Server’s response is dependent on the HTTP verb which we have used in the request.
Examples of HTTP responses
200-OK Means that the request has been completed successfully and the server has returned the response. Usually displayed after a successful GET request.
201 – Created Usually displayed after a successful POST request. It informs that the resource has been created.
400 – Bad Request Code is returned after sending a request with missing or invalid parameters. Usually displayed in reaction to POST or PUT requests.
401 – Unauthorized The code means that the user hasn´t been authorized to execute an action. For example, the user has tried to create or delete a resource without providing authentication credentials.
404 – Not Found Informs that the resource a user is looking for has not been found.
501 – Not Implemented Usually displayed when a server receives a request method it doesn’t recognize.
Endpoint and route
As we already mentioned above, the endpoint is basically an address to which we send requests. In addition, the endpoint in itself has its own parameters.
Endpoints are functions, available via API, which perform actions, for example receiving resources or creating a new user. We should say that an endpoint activates a procedure which executes a certain task. These endpoints are dependent on transmitted parameters.
For example, we can use a GET method to receive all posts: GET http://localserver/wp-json/wp/v2/posts (or testing URL from postman-echo GET https://postman-echo.com/get?foo1=bar1&foo2=bar2).
An example of using the GET method
And here, another significant term comes into play. A route for the mentioned endpoint is wp/v2/posts. The route is a way to access the endpoint. It can have multiple endpoints based on an HTTP request. The above route has the following endpoint for creating a new post: POST wp/v2/posts. This endpoint supplied by other parameters creates a new post.
To make everything clear, let’s consider the following route: wp/ v2/posts/100. This route refers to the post having an ID 100. It has the following three endpoints:
1. GET wp/v2/posts/100 It is used to retrieve the post having an ID 100.
2. PUT wp/v2/posts/100 It is used to update the post having an ID 100.
3. DELETE wp/v2/posts/100 It is used to delete the post having an ID 100.
Use in practice
Retrieving a post
To retrieve data from a server, the GET request is used. As soon as the server receives the request, it sends a collection of all objects in a form of a JSON to a client. If we want to retrieve a post, our request will look like this:
GET http://localserver/wp-json/wp/v2/posts
HOST: localserver
We can also get a specific post by mentioning its ID (in this case ID 100).
GET http://localserver/wp-json/wp/v2/posts/100
If we want to find a post according to specific criteria, we use a parameter filter[] (in this case we retrieve all posts from a category ID 1):
GET http://localserver/wp-json/wp/v2/posts?filter[cat]=1
Creating a new post
To create a new post, the POST request is used. It must include a Content-Type header set to text/xml or application/xml if you are sending an XML block, or set to application/json for a JSON request block. After the header comes the request body with data in XML or JSON that include the information for the new resource. The information in the request depends on what type of resource we are creating.
The example of a request in JSON creating a new user:
POST http://localserver/wp-json/wp/v2/users
HOST: localserver
Content-Type: application/json
{
"user": {
"name": "NewUser1",
"siteRole": "Publisher"
}
}
How to communicate with REST API in practice?
If you have already tested the work with API and want to use it in app development, you will typically use one of the libraries with the possibility of communicating in a specific programming language via HTTP protocol – for example in PHP, it is the cURL library.
For sending a request to REST API and proceeding its response, we can work on the following example of the use of cURL in PHP:
<?php
// We transmit the address of the endpoint to which we want to send a request to the function curl_init().
// The function returns a connection identifier to which we will refer by selected variable $ch.
$ch = curl_init('http://www.example.com/wp-json/wp/v2/posts');
// By the function curl_setopt() we can specify the request to the endpoint and set up a behaviour of cURL during sending a request and getting a response.
// In our case we will especially want to return the response as a result of the function curl_exec().
curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1);
// Also we will want only a body of response without http headers.
curl_setopt($ch, CURLOPT_HEADER, 0);
// If everything goes well and the server responds, the function curl_exec() will return the response.
$result = curl_exec($ch);
// We close the phrase.
curl_close($ch);
// We have the server response in the variable $result (in the case of WordPress REST API, it is in JSON).
// For further processing, we have to transfer the data in JSON format to the native PHP array or object. We will use the function json_decode().
$data = json_decode($result);
Are you already familiar with the REST API in WordPress or are there anything unclear? Write to us and we will be happy to help. In the next part of the series, you can deepen the knowledge – in a few steps we will explain how to create an application for publishing and categorizing unreleased posts using the REST API.